Maps Plugin
The meteoblue Maps Plugin makes it easy to integrate meteoblue map tiles into any website. The Tile API generates tiles that can directly be used by map libraries like Mapbox GL JS or Leaflet. Displaying weather data usually requires a time selection and a menu to select different weather variables. The meteoblue Maps Plugin saves the effort of implementing a user-interface for basic map interaction.
The Maps Plugin is initialized with an Inventory JSON that can be customized to change every aspect of a map. An Inventory JSON will be supplied by the Inventory API. Breaking changes to the specifications will then be updated transparently.
The Maps Plugin can be integrated with a couple lines of code.
Example:
<!-- Load the Mapbox GL JS mapping library from meteoblue servers -->
<script src="https://static.meteoblue.com/cdn/mapbox-gl-js/v1.11.1/mapbox-gl.js"
integrity="sha512-v5PfWsWi47/AZBVL7WMNqdbM1OMggp9Ce408yX/X9wg87Kjzb1xqpO2Ul0Oue8Vl9kKOcwPM2MWWkTbUjRxZOg=="
crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://static.meteoblue.com/cdn/mapbox-gl-js/v1.11.1/mapbox-gl.css"
integrity="sha512-xY1TAM00L9X8Su9zNuJ8nBZsGQ8IklX703iq4gWnsw6xCg+McrHXEwbBkNaWFHSqmf6e7BpxD6aJQLKAcsGSdA=="
crossorigin="anonymous">
<!-- Load the meteoblue Maps Plugin -->
<script src="https://static.meteoblue.com/lib/maps-plugin/v0.x/maps-plugin.js"></script>
<!-- Styles -->
<style>
#mapContainer {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
}
</style>
<!-- The container for the map -->
<div id="mapContainer"></div>
<script>
// Initialize the Mapbox GL JS map inside the mapContainer element.
// More configuration options for Mapbox GL JS can be found at https://docs.mapbox.com/mapbox-gl-js/api/map/#map-parameters
// Do not set the style parameter. It will be overwritten by the meteoblue Maps Plugin.
mapboxMap = new mapboxgl.Map({
container: 'mapContainer',
center: [7, 47],
zoom: 5,
hash: 'coords',
attributionControl: false,
keyboard: false
});
const lang = "en";
const apiKey = "<your_api_key>";
const maps = "windAnimation,temperature,precipitationDaily,evapotranspiration,soilMoisture,potentialEvapotranspiration,soilTemperature,humidity";
// The URL to the Inventory JSON. In this case the meteoblue API will generate one with some specified maps.
const inventoryUrl = `https://maps-api.meteoblue.com/v1/map/inventory/filter?lang=${lang}&maps=${maps}&apikey=${apiKey}`;
// Initialize the meteoblue Maps Plugin. It will generate a user interface on top of the Mapbox GL JS map according to the inventory JSON.
new meteoblueMapsPlugin({
mapboxMap: mapboxMap,
inventory: inventoryUrl,
apiKey: apiKey,
});
</script>
The code above will create a map as shown in the following screenshot.
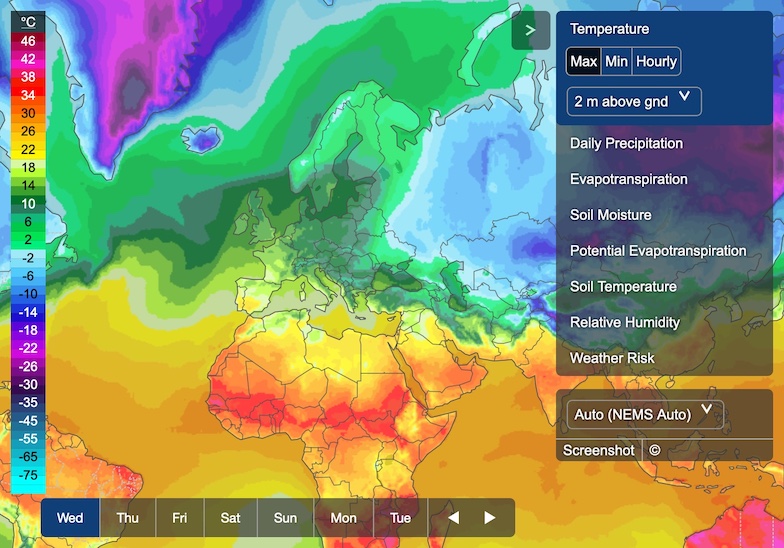
Supported Mapbox GL JS Versions
The meteoblue Maps Plugin requires Mapbox GL JS 1.6.0 or later. Version 2 (tested up to 2.8.2) is supported, but since it requires a Mapbox token, using version 1 might make more sense.
Initialisation Options
Example:
new meteoblueMapsPlugin(options: Object)
Parameter | Description |
---|---|
options.mapboxMap | Reference to a Mapbox GL JS map. |
options.inventorystring Required | URL to a inventory JSON. |
options.apiKeystring Required | API key for tile calls. |
options.bufferboolean Default: true | With buffer enabled the interface will load the map for the next time step in the background. This feature is experimental. |
options.arrowKeysboolean Default: false | Select the next/previous time step with the keyboard arrow keys. |
options.hashboolean Default: false | If true, the currently selected map and other information will be stored in the URL hash. Useful, for example, if the user shares the URL on social media. |
options.timezoneOffsetInSecondsnumber Default: 0 | Time zone offset in seconds. |
options.timezoneAbbreviationstring Default: "UTC" | Time zone abbreviation to be displayed.
Use |
options.languagestring Default: "en" | The UI language, e.g. for the time selection. This does not affect the menu language, which is set in the Inventory API URL. |
options.directionstring Default: "ltr" | The writing direction. E.g. use "rtl" for Arabic script. |
options.autoplayboolean Default: false | Automatically start playing. Applies only to maps which can be played. |
options.showSidebarboolean | "auto" Default: "auto" | Show or hide the sidebar (menu).
Use |
options.showTimeSelectionboolean Default: true | Show or hide time selection. |
options.showLegendboolean Default: true | Show or hide legend. |
options.showLoadingTimeboolean Default: false | Show loading/rendering time below loading spinner. |
options.screenshotLogoPathstring Optional | Path to logo image displayed on the screenshot. |
options.defaults.map | The initial map to be shown. |
options.defaults.variant | The initial map variant to be shown. |
options.defaults.domain | The initial domain to be used. |
options.defaults.level | The initial height level to be used. |
options.defaults.overlays | The initially enabled overlays. |
options.canUseRestrictedboolean Default: false | User can use restricted maps, e.g. paid maps. |
options.restrictedNotice.content | Message to show when a map is restricted and user options.canUseRestricted is false. |
options.restrictedNotice.actionLabelstring Optional | Label for the action button in the restricted notice. |
options.restrictedNotice.actionFunction: () => void Optional | Function to be attached to action button. |
options.customMenu | Array of menu entry labels and functions or strings.
Possible strings are {label: string, action: function | string} Example: [{ label: "Take screenshot", action: "screenshot" }, { label: "Say hi", action: () => meteoblueMaps.openModal({ content: "hi" }) }] |
options.searchBar.show boolean | Enable the search bar |
options.searchBar.placeHolderTextstring optional | Alternative text for the search bar placeholder. Useful for providing translations. |
options.searchBar.noResultsTextstring optional | Alternative text when no results matching the search are found. Useful for providing translations. |
options.controls.toggleMenuboolean Default: true | Show toggle menu button. |
options.controls.zoomboolean | string Default: "auto" | Show/hide zoom button. When set to "auto", buttons are hidden on small screens. |
options.content.topHTMLElement Optional | Element to show in the top-left corner, e.g. a logo. |
options.content.mainHTMLElement Optional | Element to show on the map. |
options.content.aboveMenuHTMLElement Optional | Element to show above the menu. |
options.content.belowMenuHTMLElement Optional | Element to show below the menu. |
options.content.belowOptionsHTMLElement Optional | Element to show below the options. |
Methods
getMap()
Get the currently selected map.
Return:
map
: (Weather) map object from the inventory
getVariant()
Get the currently selected map variant.
Return:
variant
: Variant object from the inventory
getOverlays()
Get the currently active overlays. This includes the selected overlays and the default overlays.
Return:
overlays[]
: An array of overlay objects from the inventory
getLevel()
Get the currently selected height level.
Return:
level
: Level object from the inventory
setMap(mapId) or setMap(options)
Displays the specified map. Accepts a single string or an options object to set map, variant, domain and level individually or in one go.
Parameters
mapId
(string): The ID of the map
or an object with these properties:
map?
(string): The ID of the map. Defaults to the currently selected map.variant?
(string): The ID of the variant. Defaults to the previously selected variant or the first variant.domain?
(string): The ID of the domain. Defaults to the currently selected domain or to "auto".level?
(string): The ID of the level. Defaults to the previously selected level or the first height level.
Examples:
// Set the map with ID "precipitation"
meteoblueMaps.setMap("precipitation")
// Select the map with ID "precipitation"
meteoblueMaps.setMap({ map: "precipitation" })
// Select the map with ID "precipitation" and the variant "hourly"
meteoblueMaps.setMap({ map: "precipitation", variant: "hourly" })
// Select map with ID "precipitation", variant "hourly" and height level "850mb"
meteoblueMaps.setMap({ map: "precipitation", variant: "hourly", level: "850mb" })
// Set the height level with ID "850mb"
meteoblueMaps.setMap({ level: "850mb" })
getDate()
Gets the currently selected date in UTC.
Return:
- Date
string
in ISO 8601 format if time type isregular
- timecategory object if time type is
categories
- timestep object if time type is
timesteps
undefined
for maps without time selection
Example:
const date = meteoblueMaps.getDate()
console.log(date) // "2020-11-30T09:00:00+00:00"
setDate(date)
Sets the specified date.
Parameters:
date
(string | number): If time type isregular
, date in ISO 8601 format or UNIX timestamp in milliseconds. Timezone information in ISO 8601 format will be dismissed.date
(string): If time type iscategories
, then time category value (ISO 8601 date string).date
(string): If time type istimesteps
, date in timestep format.
Examples:
meteoblueMaps.setDate("2020-03-08T12:00")
meteoblueMaps.setDate(1583667468000)
getTimeInfo()
Return:
The time info object from the inventory,
including e.g. the first and last available date.
Time type url
will always be resolved to its final type.
Example:
const timeInfo = meteoblueMaps.getTimeInfo()
console.log(timeInfo)
/*
{
"lastUpdate":"2020-11-18T09:32:02",
"interval":3600,
"type":"regular",
"last":"2020-11-25T12:00",
"current":"2020-11-18T12:00",
"first":"2020-11-18T00:00"
}
*/
getDomain()
Return:
The currently selected domain object.
Example:
const domain = meteoblueMaps.getDomain()
console.log(domain)
/*
{
"id": "NEMSGLOBAL",
"name": "NEMSGLOBAL Global",
"levels": [
{
"name": "10 m above gnd",
"id": "10 m above gnd"
},
{
"name": "80 m above gnd",
"id": "80 m above gnd"
},
...
]
}
*/
enableOverlay(overlayId)
Displays an overlay from the inventory on the map.
Parameters:
overlayId
(string): The ID of the overlay.
disableOverlay(overlayId)
Removes an overlay from the map.
Parameters:
overlayId
(string): The ID of the overlay.
getInventory()
Return:
The inventory object.
openModal(options)
Opens a modal window. Close with modal.closeModal()
.
Parameters:
options.content
(HTML element): The modal content.options.confirmLabel?
(string): Label of the confirm button. Defaults to "OK".options.onConfirm?
(function: (event) => void): Function attached to confirm button.options.closeLabel?
(string): Label of the confirm button. Defaults to "Close".options.onClose?
(function: (event) => void): Function attached to confirm button.options.title?
(string): The title.options.closeable?
(boolean): Click on X or background mask closes the modal.
Example:
const modal = meteoblueMaps.openModal({
content: "Hello, World!",
onConfirm: function () {
// do something...
modal.closeModal()
}
})
showTimeSelection()
Show the time selection.
hideTimeSelection()
Hide the time selection.
on(type, listener)
Adds a listener for events of a specified type.
Parameters:
type
(string): The event type to listen for. See "Events" section.listener
(function): The function to be called when the event is fired.
Example:
const menuChangeListener = function (event) {
console.log("User selected map with name " + event.map.name)
// User selected map with name Temperature
}
meteoblueMaps.on('menuchange', menuChangeListener)
off(type, listener)
Removes an event listener previously added with on()
.
Parameters:
type
(string): The event type to listen for. See "Events" section.listener
(function): The function previously installed as a listener.
Example:
meteoblueMaps.off('menuchange', menuChangeListener)
Events
load
Fired immediately after inventory have been downloaded an UI has been created.
Example:
const loadListener = function () {
const map = meteoblueMaps.getMap()
}
meteoblueMaps.on('load', loadListener)
menuchange
Fired when the map, variant, domain, level or overlay was changed.
Properties:
map
(object): The selected map.variant
(object): The selected map variant.domain
(object): The selected domain.level
(object): The selected height level.overlays
(object): The selected overlays.
Example:
const menuChangeListener = function (event) {
console.log("Selected map " + event.map.name + ", variant " + event.variant.name + ", level " + event.level.name)
// Selected map with name Temperature, variant daily, level 850mb
}
meteoblueMaps.on('menuchange', menuChangeListener)
datechange
Fired when the date was changed.
Properties:
date
(string): ISO 8601 or timesteps date string.
unitchange
Fired when the unit is changed.
Properties:
unit
(string): The selected unit, e.g. "C"unitType
(string): The selected unit type, e.g. "temperature"
Example:
const unitChangeListener = function (event) {
console.log("Changed " + event.unitType + "unit to " + event.unit)
// Changed temperature unit to C
}
meteoblueMaps.on('unitchange', unitChangeListener)
Custom UI Styling
Modify colours and other style attributes of the plugin through CSS variables.
Example:
<style>
:root {
--meteoblue-mapsplugin-background-primary: rgba(226, 226, 226, 0.7);
--meteoblue-mapsplugin-background-highlight: rgba(255, 255, 255, 0.5);
--meteoblue-mapsplugin-background-highlight-secondary: rgba(255, 255, 255, 0.9);
--meteoblue-mapsplugin-color-primary: rgb(0, 0, 0);
--meteoblue-mapsplugin-text-shadow: 0 0 5px rgb(255, 255, 255);
}
</style>
Available CSS Variables
- --meteoblue-mapsplugin-background-primary
- --meteoblue-mapsplugin-background-highlight
- --meteoblue-mapsplugin-background-highlight-secondary
- --meteoblue-mapsplugin-color-primary
- --meteoblue-mapsplugin-color-progress-bar
- --meteoblue-mapsplugin-color-link-hover
- --meteoblue-mapsplugin-color-toggle
- --meteoblue-mapsplugin-color-scrollbar
- --meteoblue-mapsplugin-border
- --meteoblue-mapsplugin-border-radius
- --meteoblue-mapsplugin-text-shadow
- --meteoblue-mapsplugin-icon-arrow
- --meteoblue-mapsplugin-icon-close
- --meteoblue-mapsplugin-icon-play
- --meteoblue-mapsplugin-icon-stop
- --meteoblue-mapsplugin-icon-menu
Recommended use cases and limitations
The maps plugin is particularly suitable as plug-and-play solution. It offers a fair amount of customizability and flexibility. However, some parts, especially the animations for wind, satellite and radar, take over full control of the canvas, which can make it hard to add further layers or background maps. Also, it is not always guaranteed to support the latest version of Mapbox GL JS. Since Mapbox GL JS version 2 requires a Mapbox token, we recommend using version 1. The maps plugin is a great choice as an easy to implement drop-in for new or existing applications and if you need the animations for wind, satellite and radar.
If you already have an existing mapping solution, want full flexibility or prefer self-hosted code, use our Tile API or Satellite and Radar Tile API instead.
For the most easy-to-use solution, use our free Widget Maps.
Hillshades
Unlike the maps on meteoblue.com, the Maps Plugin does not include hillshades. Please contact us for further information on how to obtain a licence for hillshades.
Maps Plugin FAQ
Where are the units of measurement set?
The units of measurement (Celsius, Fahrenheit, km/h, mph, ...) are set in the Inventory API URL.
How to add a location marker?
The Maps Plugin is just a plugin for Mapbox GL JS, which means that all features of Mapbox GL JS can be used. For adding a location marker, please refer to https://docs.mapbox.com/mapbox-gl-js/example/add-a-marker/.